Mastering Wireless Connectivity for Arduino: A Comprehensive Guide to Adding Wi-Fi and Bluetooth
Learn how to add Wi-Fi and Bluetooth to your Arduino projects with this comprehensive guide. Discover the best hardware, step-by-step tutorials, and tips to create powerful IoT and smart home applications.
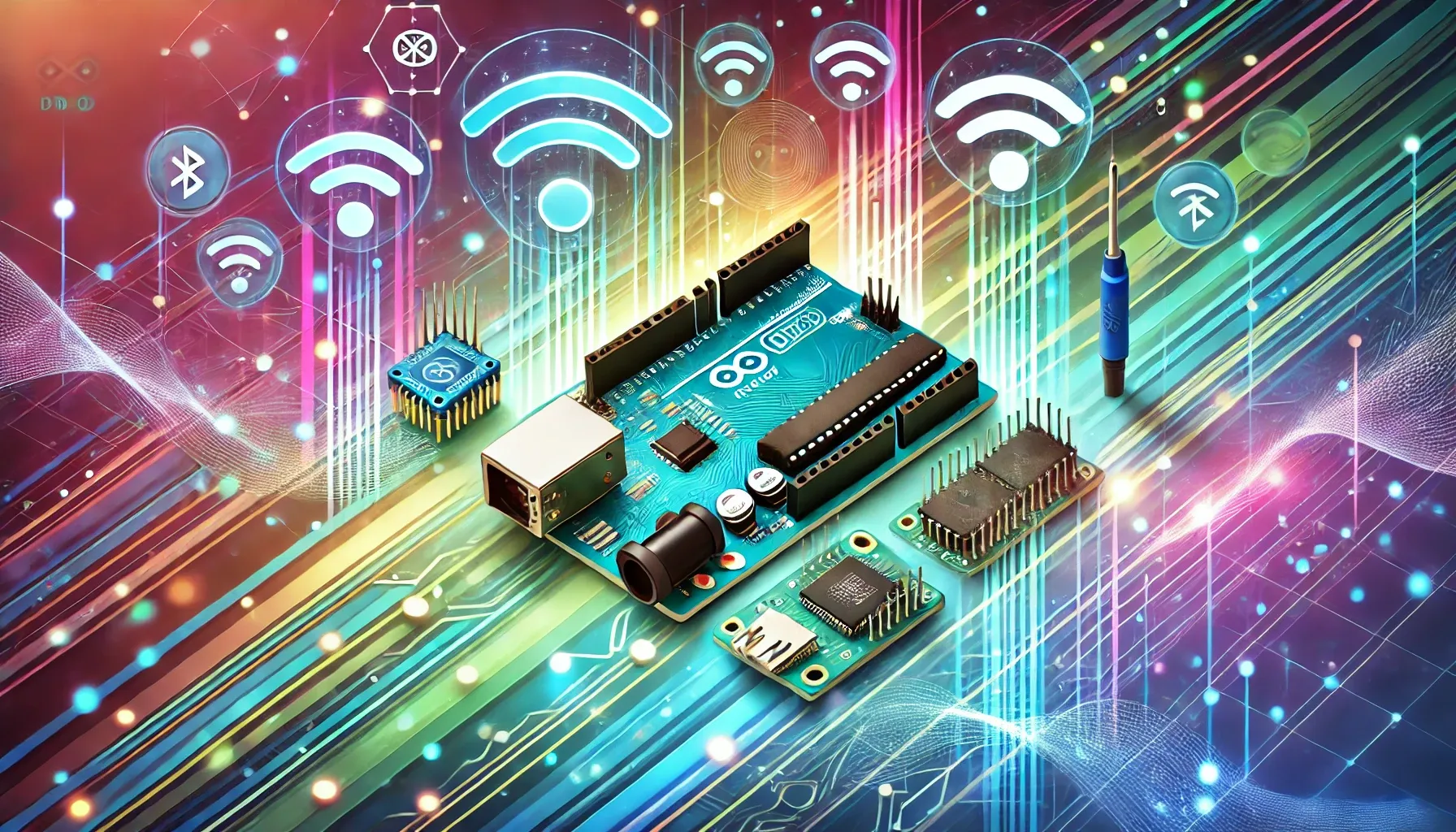
Arduino projects have revolutionized the DIY electronics space, allowing hobbyists and professionals alike to create powerful, innovative solutions. But to take your Arduino project to the next level, adding wireless connectivity—be it Wi-Fi, Bluetooth, or both—is often essential. Wireless features open up possibilities for IoT (Internet of Things) projects, remote control systems, and data-driven applications.
This guide will take you through the process of adding Wi-Fi and Bluetooth to your Arduino projects, breaking down the concepts, hardware options, and steps to get started. Whether you're a beginner or an experienced tinkerer, this article will help you integrate wireless connectivity seamlessly.
Understanding Wireless Connectivity: Why Wi-Fi and Bluetooth Matter
Wireless connectivity enables devices to communicate without physical connections, expanding functionality and convenience. Here’s why adding Wi-Fi or Bluetooth to your Arduino project is a game-changer:
- Wi-Fi: Ideal for IoT applications, Wi-Fi allows your Arduino to connect to the internet, enabling real-time data sharing, cloud integration, and remote control.
- Bluetooth: Perfect for short-range communication, Bluetooth is energy-efficient and well-suited for wearable devices, home automation, and app-controlled gadgets.
When deciding which wireless protocol to use, consider the range, power consumption, and the intended use of your project.
Choosing the Right Hardware for Wireless Connectivity
Adding Wi-Fi or Bluetooth to your Arduino project requires compatible hardware. Here are the most common options:
Wi-Fi Modules
- ESP8266: A budget-friendly, versatile module that supports Wi-Fi connectivity. It can operate as a standalone microcontroller or be paired with an Arduino board.
- ESP32: A more powerful alternative to the ESP8266, featuring both Wi-Fi and Bluetooth capabilities, making it a popular choice for advanced projects.
- Arduino MKR Wi-Fi 1010: Designed for IoT projects, this board integrates Wi-Fi functionality and is beginner-friendly.
Bluetooth Modules
- HC-05/HC-06: Affordable and widely used, these modules are great for basic Bluetooth communication.
- HM-10: A BLE (Bluetooth Low Energy) module that consumes less power and supports modern devices.
- Arduino Nano 33 BLE: A compact board with built-in BLE capabilities, ideal for space-constrained projects.
Shields and Add-ons
For standard Arduino boards like the Uno, shields such as the Arduino Wi-Fi Shield or Bluetooth Shield simplify the process of adding wireless connectivity.
Preparing Your Arduino for Wireless Integration
Before diving into wireless connectivity, ensure your Arduino is set up and ready. If you're new to Arduino, check out our beginner's guide to Arduino for setup instructions.
Essential Components
- Arduino board
- Wireless module or shield
- Breadboard and jumper wires
- External power supply (if needed)
- Laptop/PC with Arduino IDE installed
Libraries and Drivers
To work with Wi-Fi or Bluetooth modules, you’ll need specific libraries. For example:
- Wi-Fi: ESP8266WiFi or WiFi.h
- Bluetooth: SoftwareSerial or BLEPeripheral.h
Install these libraries through the Arduino IDE’s Library Manager for seamless integration.
Adding Wi-Fi to Your Arduino Project
Step 1: Connecting the Hardware
- Attach the Wi-Fi module (e.g., ESP8266) to your Arduino. Use jumper wires to connect:
- VCC to 3.3V or 5V (as per the module's specs)
- GND to GND
- TX to RX
- RX to TX
- If using a shield, simply plug it onto the Arduino board.
Step 2: Programming the Module
Use the Arduino IDE to write a sketch that enables Wi-Fi functionality. For instance, connect your Arduino to a local Wi-Fi network:
#include <ESP8266WiFi.h>
const char* ssid = "Your_SSID";
const char* password = "Your_Password";
void setup() {
Serial.begin(115200);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting...");
}
Serial.println("Connected!");
}
void loop() {
// Add your code here
}
Step 3: Testing the Connection
Open the Serial Monitor in the Arduino IDE to verify the connection status. Once connected, you can expand the code to include HTTP requests, MQTT communication, or real-time data monitoring.
Adding Bluetooth to Your Arduino Project
Step 1: Setting Up the Hardware
- Connect the Bluetooth module (e.g., HC-05) to the Arduino:
- VCC to 5V
- GND to GND
- TX to RX
- RX to TX
- Some modules require configuring the baud rate and mode via AT commands before use.
Step 2: Writing the Sketch
Here’s a simple sketch to enable Bluetooth communication:
#include <SoftwareSerial.h>
SoftwareSerial BTSerial(10, 11); // RX, TX
void setup() {
Serial.begin(9600);
BTSerial.begin(9600); // Match the baud rate of your module
Serial.println("Bluetooth is ready!");
}
void loop() {
if (BTSerial.available()) {
char c = BTSerial.read();
Serial.write(c);
}
if (Serial.available()) {
char c = Serial.read();
BTSerial.write(c);
}
}
Step 3: Pairing and Testing
Pair the Bluetooth module with your smartphone or PC and use a terminal app to send and receive data. Test the setup by sending simple text commands.
Common Challenges and Troubleshooting
Wireless connectivity can be tricky. Here are some tips for troubleshooting:
- Connection Issues:
- Double-check wiring and power supply.
- Ensure correct SSID and password for Wi-Fi or pairing code for Bluetooth.
- Unstable Connections:
- Use decoupling capacitors to stabilize the power supply.
- Avoid noisy environments that may interfere with signals.
- Code Errors:
- Verify library versions and update them if needed.
- Use debug messages in your code to identify problem areas.
Pro Tips for Wireless Arduino Projects
- Optimize Power Usage: Use sleep modes or BLE for battery-powered projects.
- Plan Network Topology: For Wi-Fi projects, ensure your network can handle multiple devices.
- Leverage Open-Source Tools: Platforms like Node-RED or MQTT enhance project capabilities.
For more programming tips, check out our guide to writing Arduino sketches.
Conclusion: Expanding Your Arduino Projects with Wireless Connectivity
Adding Wi-Fi and Bluetooth to your Arduino projects transforms simple DIY builds into powerful, connected systems. From smart homes to IoT devices, the possibilities are endless. With the right hardware, libraries, and code, you can bring your ideas to life and explore the vast world of wireless Arduino applications.
If this guide inspired you, share your projects or challenges in the comments below. Let’s create something amazing together!
Resources for Arduino Wireless Projects
To successfully integrate Wi-Fi and Bluetooth into your Arduino projects, it’s essential to have access to reliable tools and community support. Here are some valuable resources to guide you:
Official Arduino Resources
- Arduino Official Documentation: Comprehensive setup guides and tutorials for all Arduino boards.
- Arduino Forums: A vibrant space for asking questions, sharing projects, and finding expert advice on all things Arduino.
- Arduino Libraries: A repository of libraries to expand Arduino's capabilities, including Wi-Fi and Bluetooth.
Wi-Fi Modules Documentation
- ESP8266 Official Documentation: Datasheets, setup guides, and more for ESP8266.
- ESP32 Official Documentation: Detailed information on ESP32’s Wi-Fi and Bluetooth features.
Bluetooth Module Resources
- HC-05 AT Command Set: Learn how to configure HC-05 modules using AT commands.
- HM-10 BLE Documentation: An overview of BLE functionality and setup for HM-10.
Community Forums and Support
- ESP32 Community Forum: Engage with fellow enthusiasts to troubleshoot and share ideas for ESP32 projects.
- ESP8266 Community Forum: A hub for discussions and solutions for ESP8266 projects.
- Reddit r/Arduino: Join a thriving community of Arduino users on Reddit.
Additional Tools and Resources
- Fritzing: A tool for designing circuit schematics and diagrams.
- Tinkercad Circuits: A beginner-friendly online circuit simulation tool.
- Binary Tech Labs Blog: Explore tutorials and guides, including Getting Started with Arduino and Learn Arduino Programming.
- YouTube Channels: Search for Arduino tutorials and project ideas.
💡 Important Disclosure
This article contains affiliate links, which means I may earn a small commission if you click through and make a purchase—at no additional cost to you. These commissions help support the ongoing creation of helpful content like this. Rest assured, I only recommend products and services I personally use or genuinely believe can provide value to you.
Thanks for Your Support!
I truly appreciate you taking the time to read my article. If you found it helpful, please consider sharing it with your friends or fellow makers. Your support helps me continue creating content like this.
- Leave a Comment: Got questions or project ideas? Drop them below—I'd love to hear from you!
- Subscribe: For more tutorials, guides, and tips, subscribe to my YouTube channel and stay updated on all things tech!
- Shop & Support: If you're ready to get started, check out the recommended products in my articles using my affiliate links. It helps keep the lights on without costing you anything extra!
Thanks again for being part of this community, and happy building!