Streamline Your Workflow: A Hands-On Guide to Containerizing Projects with Docker
Learn how to containerize your projects using Docker with this step-by-step guide. From understanding the basics of Docker to crafting efficient Dockerfiles and managing containers, this article equips you with practical skills to streamline your development workflow.
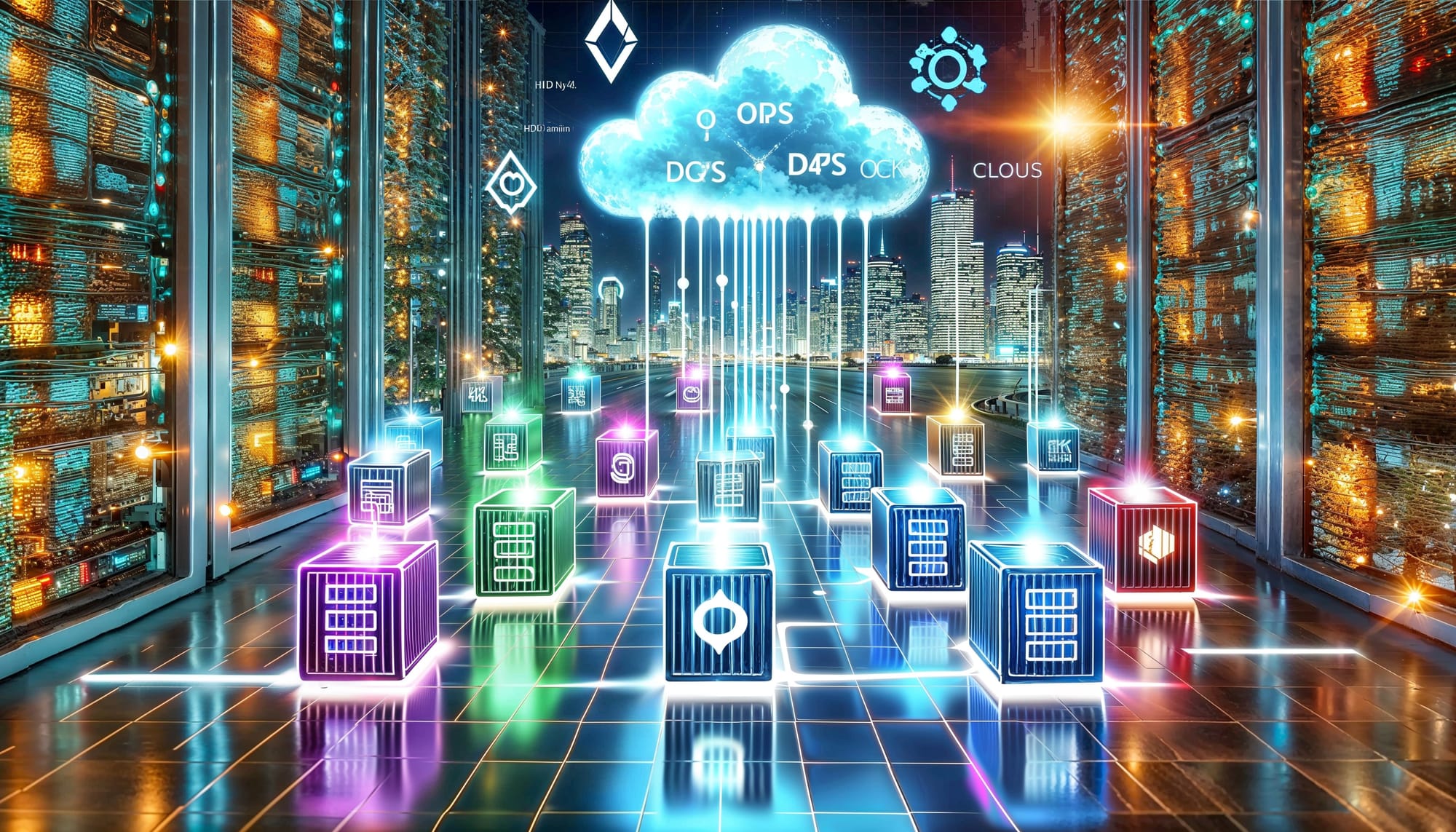
Why Containerization Matters in Modern Development
Imagine this: you’ve spent weeks perfecting a project on your machine. It runs flawlessly, handles edge cases with ease, and meets every requirement. But when it’s time to deploy it to production or share it with your team, chaos ensues. Dependencies conflict, libraries are missing, and the environment behaves differently than expected. This scenario is far too common in software development, but it doesn’t have to be. Enter Docker—a tool that eliminates these headaches by packaging your application and its dependencies into a single, portable unit called a container.
Containerization has revolutionized how developers build, ship, and run applications. Unlike traditional virtual machines, which emulate entire operating systems, containers share the host system’s kernel while isolating processes, making them lightweight and efficient. Docker, as the leading containerization platform, simplifies this process by providing a consistent runtime environment across all stages of development. Whether you’re working on a small personal project or a large-scale enterprise application, Docker ensures that your code behaves the same way everywhere, on your laptop, in testing environments, and most importantly on your production servers.
The benefits don’t stop there. Containers allow for faster deployment cycles, easier scaling, and seamless collaboration between teams. They also integrate effortlessly with modern DevOps practices like continuous integration and continuous delivery (CI/CD). By adopting Docker, you’re not just solving immediate problems; you’re future-proofing your workflow. In the following sections, we’ll dive into how Docker works under the hood and guide you step-by-step through containerizing your own projects.
Understanding Docker: The Basics
At its core, Docker operates on two foundational concepts: images and containers. Think of an image as a blueprint or snapshot of your application and its environment. It contains everything needed to run your software; your code, runtime libraries, system tools, and configurations, all bundled together in a standardized format. A container, on the other hand, is a running instance of that image. When you “spin up” a container, Docker uses the image to create an isolated environment where your application can execute (live) without interference from the host system or other containers.
Docker achieves this isolation through a combination of Linux namespaces and control groups (cgroups). Namespaces ensure that each container operates in its own isolated space, preventing conflicts between processes, file systems, and network interfaces. Meanwhile, cgroups manage resource allocation, ensuring that no single container monopolizes CPU, memory, or disk usage. Together, these mechanisms make containers both secure and efficient.
The Docker Engine serves as the backbone of this system. It consists of a daemon process that manages containers, a REST API for interacting with the engine programmatically, and a command-line interface (CLI) that allows developers to interact with Docker directly. When you issue commands like docker build
or docker run
, the CLI communicates with the daemon, which then executes the requested operations.
This architecture offers several advantages over traditional virtual machines (VMs). While VMs require a full guest operating system for each instance, Docker containers share the host OS kernel, drastically reducing overhead. This means containers start faster, consume fewer resources, and scale more efficiently. Additionally, because containers package only what’s necessary to run an application, they’re inherently portable. You can move them between different environments—development laptops, testing servers, cloud platforms—without worrying about compatibility issues.
By leveraging Docker’s layered filesystem, images are built incrementally, reusing existing layers whenever possible. This not only speeds up builds but also minimizes storage requirements. For example, if two images share the same base layer (like a common Linux distribution), Docker will store that layer once and reference it in both images. These efficiencies make Docker an indispensable tool for modern development workflows.
Getting Started with Docker: Installation and Setup
Before diving in, you’ll need to install Docker on your system. The installation process varies slightly depending on your operating system, but Docker provides clear, step-by-step instructions for each platform. On Windows and macOS, Docker Desktop is the recommended option, offering a user-friendly interface alongside powerful CLI tools. For Linux users, Docker can be installed via package managers like apt
for Ubuntu or yum
for CentOS. Regardless of your OS, the official Docker documentation is an excellent resource to guide you through the setup.
Once Docker is installed, verify that it’s running correctly by opening a terminal or command prompt and entering docker --version
. This command should return the version number of Docker installed on your system. To confirm Docker is operational, try running a simple test container with docker run hello-world
. If everything is configured properly, Docker will pull the "hello-world" image from its repository, create a container, and display a success message.
With Docker up and running, the next step is to familiarize yourself with some essential commands. Start by exploring the docker ps
command, which lists all currently running containers. Adding the -a
flag (docker ps -a
) shows both active and stopped containers, giving you a complete overview of your environment. To view available images on your system, use docker images
. This command displays details such as image names, tags, and sizes, helping you manage your resources effectively.
Another critical command is docker pull
, which retrieves images from Docker Hub, the default registry for Docker images. For example, docker pull ubuntu
fetches the latest Ubuntu image. Once downloaded, you can launch a container based on this image using docker run
. Adding flags like -it
(interactive terminal) or -d
(detached mode) modifies how the container behaves. For instance, docker run -it ubuntu bash
starts an interactive session with the Ubuntu shell, allowing you to experiment within the container.
These basic commands form the foundation of working with Docker. As you grow more comfortable, you’ll discover additional options and flags that enhance functionality. For now, mastering these essentials will prepare you to take the next step: creating your own Dockerfile and building custom images tailored to your projects.
Crafting Your First Dockerfile
Now that you’ve mastered the basics, it’s time to create your first Dockerfile—the blueprint for your custom container. A Dockerfile is essentially a script that defines how your image should be built, specifying everything from the base operating system to the final application setup. Writing an effective Dockerfile involves careful planning to ensure efficiency, maintainability, and portability. Let’s break down the key components and best practices for crafting one.
Start by selecting an appropriate base image. Base images serve as the foundation for your container and typically include a minimal operating system like Alpine Linux or Ubuntu. For example, if you’re building a Python application, you might choose python:3.10-slim
as your base image. This ensures your container includes the necessary runtime without unnecessary bloat. Specify the base image at the top of your Dockerfile using the FROM
instruction:
FROM python:3.10-slim
Next, set up the working directory within the container using the WORKDIR
instruction. This creates a consistent path for subsequent commands and helps organize your files. For instance:
WORKDIR /app
With the environment prepared, copy your application code into the container using the COPY
instruction. Be selective about what you include—only copy files essential for running your application. For example:
COPY . .
Dependencies are often the next step. Use the RUN
instruction to execute commands like installing packages or compiling assets. If you’re working with Python, you might install dependencies listed in a requirements.txt
file:
RUN pip install --no-cache-dir -r requirements.txt
To define the default command that runs when the container starts, use the CMD
instruction. This could be starting a web server, running a script, or launching an interactive shell. For example:
CMD ["python", "app.py"]
Throughout this process, keep your Dockerfile concise and modular. Avoid including unnecessary steps or redundant commands, as each instruction adds a new layer to the image. To optimize performance, group related commands together and leverage caching. For instance, installing dependencies before copying application code ensures that changes to your code won’t invalidate the dependency cache.
Finally, test your Dockerfile thoroughly. Build the image using docker build -t my-app .
and run the container with docker run my-app
. If something doesn’t work as expected, revisit your Dockerfile and refine it. With practice, you’ll develop an intuition for structuring efficient, reliable Dockerfiles that streamline your development process.
Running and Managing Containers
With your Dockerfile ready and your image built, it’s time to bring your container to life. Launching a container is straightforward, but understanding how to manage it effectively is crucial for maximizing Docker’s potential. Start by running your container with the docker run
command. For example, if your image is named my-app
, you can start it with:
docker run -d -p 8080:5000 my-app
Here, the -d
flag runs the container in detached mode, freeing up your terminal for other tasks. The -p
flag maps port 5000 inside the container to port 8080 on your host machine, allowing external access to your application. Adjust these values based on your app’s configuration and hosting requirements.
Once your container is running, monitor its status with docker ps
. This command lists all active containers, showing details like container IDs, names, and mapped ports. To inspect a specific container’s logs, use docker logs <container_id>
. Logs provide valuable insights into your application’s behavior, helping you debug issues or track performance.
Managing multiple containers requires organization. Assign meaningful names to your containers using the --name
flag during startup. For example:
docker run -d -p 8080:5000 --name my-running-app my-app
This makes it easier to reference containers later. To stop a running container, use docker stop <container_name>
or docker stop <container_id>
. Similarly, remove unused containers with docker rm
. Combine these commands to clean up stopped containers efficiently:
docker rm $(docker ps -aq -f status=exited)
For advanced scenarios, Docker Compose simplifies managing multi-container setups. Define your services, networks, and volumes in a docker-compose.yml
file, then use docker-compose up
to start everything at once. This approach is ideal for applications requiring databases, caches, or other dependencies.
By mastering these commands and strategies, you’ll gain full control over your containers, ensuring smooth operation and scalability for your projects.
Elevate Your Workflow with Docker
By now, you’ve seen how Docker transforms the way we build, deploy, and manage applications. From eliminating environment inconsistencies to streamlining collaboration, its impact is undeniable. Imagine deploying updates with confidence, knowing your application will behave exactly as expected—whether it’s running on a colleague’s laptop or a production server. That’s the power Docker puts in your hands.
But the journey doesn’t end here. The true value of Docker lies in experimentation. Try containerizing different types of projects—web apps, data pipelines, or even machine learning models. Explore Docker Hub to discover pre-built images that accelerate your work. Dive into advanced features like multi-stage builds to optimize image size or Kubernetes for orchestrating complex deployments. Each step you take deepens your understanding and expands your capabilities.
Don’t wait to unlock this potential. Start small, perhaps by containerizing a simple project, and let curiosity guide you forward. The more you use Docker, the more it becomes an indispensable part of your toolkit. Share your experiences, challenges, and successes—because every container you build brings you closer to mastering the art of modern development.
Thanks for Your Support!
I truly appreciate you taking the time to read my article. If you found it helpful, please consider sharing it with your friends or fellow makers. Your support helps me continue creating content like this.
- Leave a Comment: Got questions or project ideas? Drop them below—I'd love to hear from you!
- Subscribe: For more tutorials, guides, and tips, subscribe to my YouTube channel and stay updated on all things tech!
- Shop & Support: If you're ready to get started, check out the recommended products in my articles using my affiliate links. It helps keep the lights on without costing you anything extra!
Thanks again for being part of this community, and happy building!